go언어 따라해보기(3) - slice
2021. 12. 13. 14:15ㆍ기타
* slice 이것저것
더보기
package main
import "fmt"
func main() {
//빈 동적 배열(slice) 생성
var a []int
fmt.Println("len(a) = ", len(a))
fmt.Println("cap(a) = ", cap(a))
/*
길이 : 쓰고 있는 공간
Capacity : 확보한 공간
*/
//길이 : 5, capacity : 5 동적 배열(slice) 생성
b := []int{1, 2, 3, 4, 5}
fmt.Println("len(b) = ", len(b))
fmt.Println("cap(b) = ", cap(b))
//길이 : 0, capacity : 8 동적 배열(slice) 생성
c := make([]int, 0, 8)
fmt.Println("len(c) = ", len(c))
fmt.Println("cap(c) = ", cap(c))
c = append(c, 1)
fmt.Println("len(c) = ", len(c))
fmt.Println("cap(c) = ", cap(c))
//append시 capacity에 따라서 같은 slice가 반환될수도 있고
//복사 생성후 만들어진 다른 slice가 반환될수도 있다
d := []int{1, 2}
e := append(d, 3)
//주소번지를 출력
fmt.Printf("d : %p, e : %p", d, e)
fmt.Println()
for i := 0; i < len(d); i++ {
fmt.Printf("c[%d} : %d, ", i, d[i])
}
fmt.Println()
fmt.Println("len(d) : ", len(d), ", cap(d) : ", cap(d))
fmt.Println()
for i := 0; i < len(e); i++ {
fmt.Printf("e[%d] : %d, ", i, e[i])
}
fmt.Println()
fmt.Println("len(e) : ", len(e), ", cap(e) : ", cap(e))
fmt.Println()
//append시 Capacity가 여유가 있어서 다른 메모리를 할당받지 않으면
//같은 주소에 추가만 한다
f := make([]int, 2, 4)
g := append(f, 1)
fmt.Printf("%p %p\n", f, g)
f[0] = 1
f[1] = 2
fmt.Println(f)
fmt.Println(g)
g[0] = 4
g[1] = 5
fmt.Println(f)
fmt.Println(g)
}
더보기
package main
import "fmt"
func main() {
a := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}
//4번째 인덱스부터 6번째 인덱스 전까지
fmt.Println("a[4:6] : ", a[4:6])
//4번째 인덱스부터
fmt.Println("a[4:] : ", a[4:])
//처음부터 4번째 인덱스까지
fmt.Println("a[:4] : ", a[:4])
//b는 원 배열을 가르키는 포인터이므로
//변경하게 되면 원 배열을 변경한다
b := a[1:3]
b[0] = 0
b[1] = 1
fmt.Println("a : ", a)
//가장 뒷자리 숫자를 없애면서 그 숫자가 무엇인지 출력
var Num int
a, Num = RemoveBack(a)
fmt.Println(a, Num)
a, Num = RemoveBack(a)
fmt.Println(a, Num)
a, Num = RemoveFront(a)
fmt.Println(Num, a)
a, Num = RemoveFront(a)
fmt.Println(Num, a)
}
func RemoveBack(a []int) ([]int, int) {
return a[:len(a)-1], a[len(a)-1]
}
func RemoveFront(a []int) ([]int, int) {
return a[1:len(a)], a[0]
}
* slice는 3개의 property로 구성된 구조체이다
1) Pointer : 시작주소
2) len : 갯수
3) capacity : 최대갯수
더보기
package main
import "fmt"
func main() {
var s []int
s = make([]int, 3)
s[0] = 100
s[1] = 200
s[2] = 300
//초기 3개 생성시 갯수 3개, capcity : 3개
fmt.Println(s, len(s), cap(s))
s = append(s, 400, 500, 600, 700)
//4개 추가시 갯수 7개, capcity : 8개
fmt.Println(s, len(s), cap(s))
}
* 메모리를 공유하다 보니 문제가 발생하기도 한다
더보기
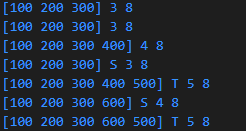
package main
import "fmt"
func main() {
var s []int
s = make([]int, 3, 8)
s[0] = 100
s[1] = 200
s[2] = 300
//초기 3개 생성시 갯수 3개, capcity : 3개
fmt.Println(s, len(s), cap(s))
t := append(s, 400)
//4개 추가시 갯수 7개, capcity : 8개
fmt.Println(s, len(s), cap(s))
fmt.Println(t, len(t), cap(t))
t = append(t, 500)
fmt.Println(s, "S", len(s), cap(s))
fmt.Println(t, "T", len(t), cap(t))
s = append(s, 600)
fmt.Println(s, "S", len(s), cap(s))
fmt.Println(t, "T", len(t), cap(t))
}
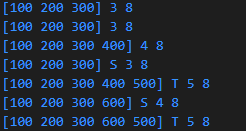
'기타' 카테고리의 다른 글
go언어 - Cross Compile (Windows -> Linux Arm) (0) | 2022.01.25 |
---|---|
[javascript] 벽돌깨기 게임 (0) | 2022.01.14 |
go - web 따라해보기(1) (0) | 2021.12.12 |
go언어 따라해보기(2) - 문자열, Swap, 구조체, 포인터, 가비지 콜렉터 (0) | 2021.12.07 |
go언어 따라해보기(1) - 표준 입출력, if, switch.. case, for, 함수사용법 (0) | 2021.12.04 |